I frequently get asked by family, friends, and acquaintances why they should use Bing over our competitors. This post is a comprehensive answer to that question, as much as it can be.
Note that I’m hardly an unbiased observer. I work for Bing, but I work deep in the layers that do the bulk of query serving, not on user-facing features. If you want a technical peek at the kind of work I typically do, you can read my book about Writing High-Performance .NET Code.
This post isn’t about that. It’s about all of the things I love about Bing. I haven’t been asked to write this. I’m doing it completely on my own, without the knowledge of people whose job it typically is to write about this kind of stuff. But I don’t see many blogs talking about these things consistently, or organizing them into a coherent list. My hope is that this will be that list, updated over time.
So standard disclaimer applies: This blog post is my opinion and may not represent those of my employer.
Second disclaimer: I will not claim that all of the things I list are unique to Bing. Some are, some aren’t, but taken together, they add up to an impressive whole, that I believe is better overall.
Third disclaimer: new features come online all the time, and sometimes features disappear. Tweaks are always being made. Some of the described features may work differently in your market, or may change in the future.
To try these examples out for yourself, you can click on most of the images below to take you to the actual results page on Bing.com.
On to the list:
1. Search Engine Result Quality
Bing’s results are every bit as relevant as Google’s and often more so. While relevance is a science that does have objective measures, I do not know of any publically available reports that compare Bing and Google with any degree of scientific precision. However, reputable sources such as SearchEngineLand have weighed in and found Bing superior in many areas.
Not too surprisingly, there was not a massive disparity in the results of my little test. In fact, Bing came out on top. Some queries performed very differently than others, for example, Bing was able to tell that my query for “Attorney Tom Brady”, was looking for an attorney and not the pictures of the hunky Patriots quarterback served up by Google.
Bing also did well with date nuances, unlike Google…
For the layperson, the relevance of a search engine is often personal and subjective. I enthusiastically recommend the BingItOn challenge that allows you to perform brand-blind search comparisons.
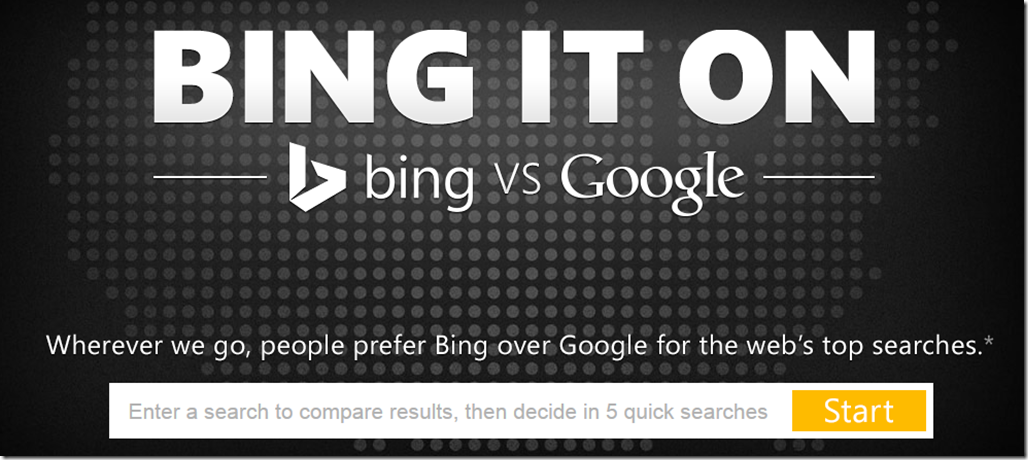
Bing certainly did not start out on an equal footing. Even when I started working at Bing over 6 years ago (before it was Bing), I would sometimes have to jump to Google to find something a little less obvious. The only reason I visit Google now is to verify the appearance of my blog and websites in their index.
2. The Home Page Photo
This was the killer feature that “launched” Bing in the minds of many people. Not just any photo, but exceptional works of art from Getty, 500px, and more. (At one time, photo submissions were accepted from Bing employees. I should see if that’s still possible…)
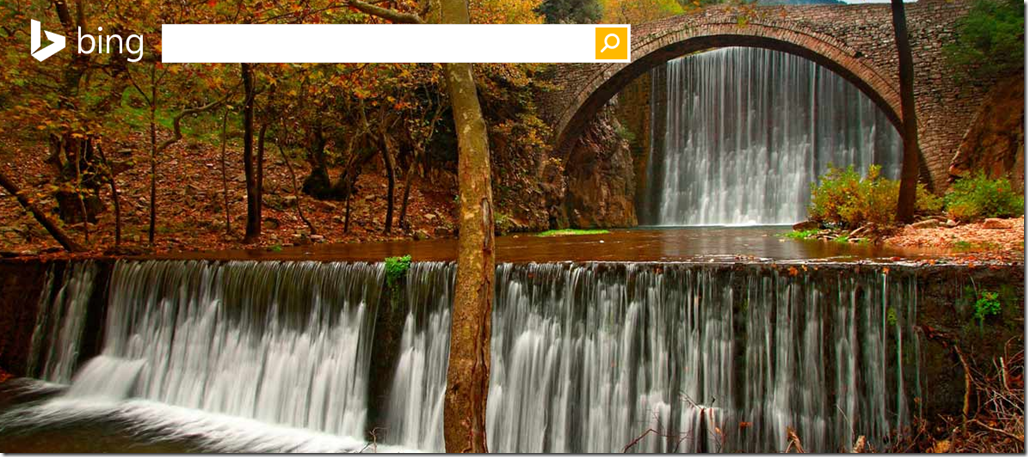
These photos showcase the beauty of our world and culture. Localized versions of Bing.com often have different photos on the same day, giving a meaningful interaction to users all over the world.
You can also view pictures from previous days.
3. Dive Into the Photo to Explore the World
In the bottom-right of the photo, there is an Info button that will take you to a search result page with more information about the subject of the photo.

In addition, as you move the mouse over the image, four different “hotspots,” marked with semi-transparent squares are highlighted. Clicking on them takes you to search results pages with more information about related topics, such as the location, similar topics, and more.
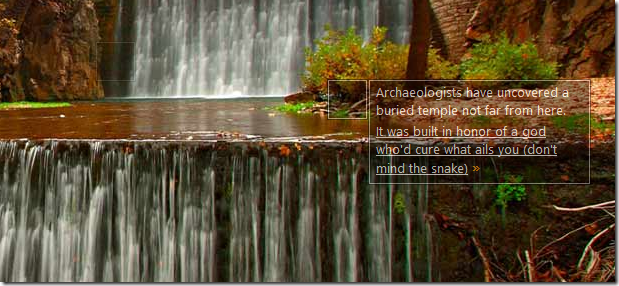
4. Use the Photo as your Windows Desktop Wallpaper

Click on the Download button in the lower-right corner to download the image to your computer for use as your wallpaper. A light Bing watermark will be embedded.
5. See Every Amazing Photo from the last 5 Years
Just visit the Bing Homepage Gallery and view every photo in one list, or filter them to try to find your favorites.
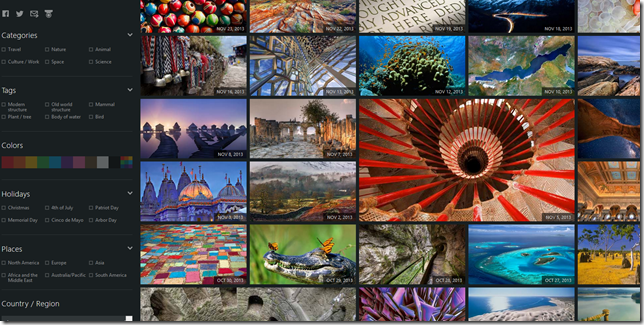
6. Bing Desktop Brings it all to your Windows Desktop
Bing Desktop takes that gorgeous photo and automatically applies it as your Windows Desktop wallpaper image each day. From the floating toolbar or the task bar, it provides instant access to searching Bing or your entire computer, including inside documents. Bing Desktop also shows you feeds of news, photos, videos, weather, and your Facebook news feed. All of this is configurable. You could use it just to change the wallpaper if you like.
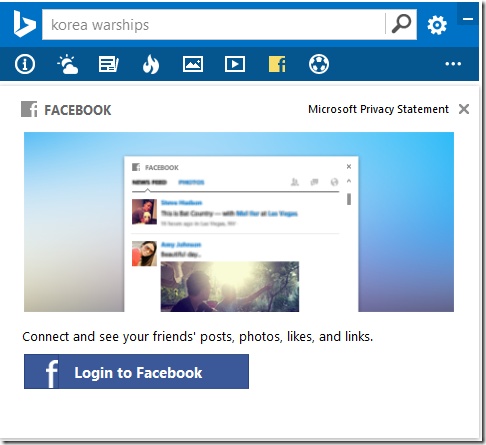
7. More Attractive Results Page
Beauty is in the eye of the beholder, but I don’t know if anyone can look at Google’s search results page and claim they’re attractive, especially when laid next to Bing’s. It’s true for nearly all results, but especially true for product searches, technical queries, and many other structured-data type results.
Here is one for a camera. Bing breaks out a bunch of information and review sources on the right, which is already a great head start, but even in the normal web results in the main part of the page, Bing has an edge. The titles, links, and subheadings with ratings all look better.
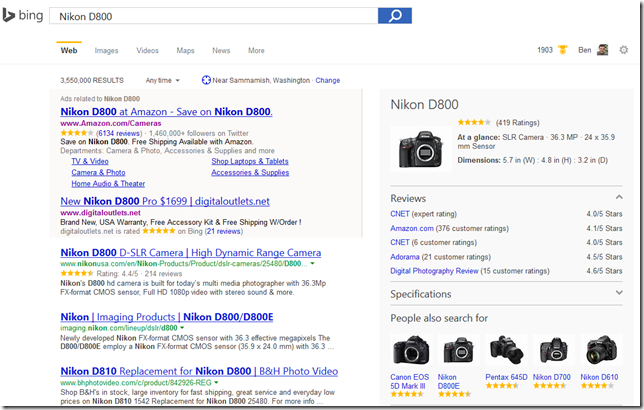
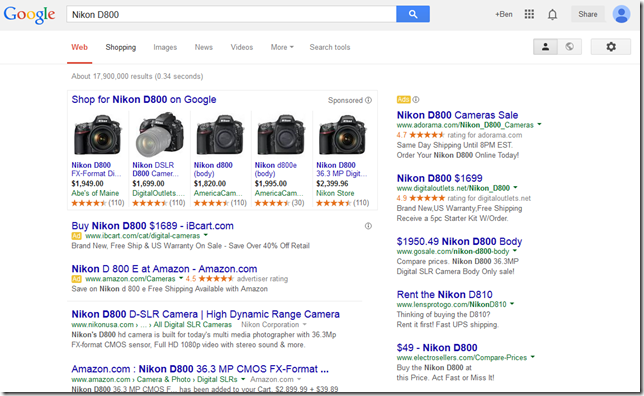
Try it out on a bunch of different types of queries. Bing just looks better, in addition to giving great results.
8. Freedom to Innovate
Bing tries a lot of experiments, throws a lot of new features out there to see what works and what doesn’t. We have that freedom to try all sorts of new things that more established players may not enjoy. Bing can change its look and functionality drastically over time to attract new types of users. You will see a lot of new things on Bing if you start paying attention. As a challenger, we are less beholden to advertisers then other search engines.
9. News Carousel
Along the home page photo is a carousel of topics, including top news (customizable to topics you are interested in), weather, trending searches, and more. This list is related to your Cortana entries as well (more on Cortana later).

You can collapse this bar in two stages. The first click will remove pictures and reduce the number of headlines.

A second click will remove all traces of it, other than the button bringing it back.

10. Image Search
Bing’s image search functionality is unparalleled. It presents related searches as well as a ton of filtering mechanisms in an attractive, compact grid that maximizes the screen real estate so you can find what you need faster. Bing also removes duplicates from this list so it doesn’t end up being just a long selection of the exact same image.
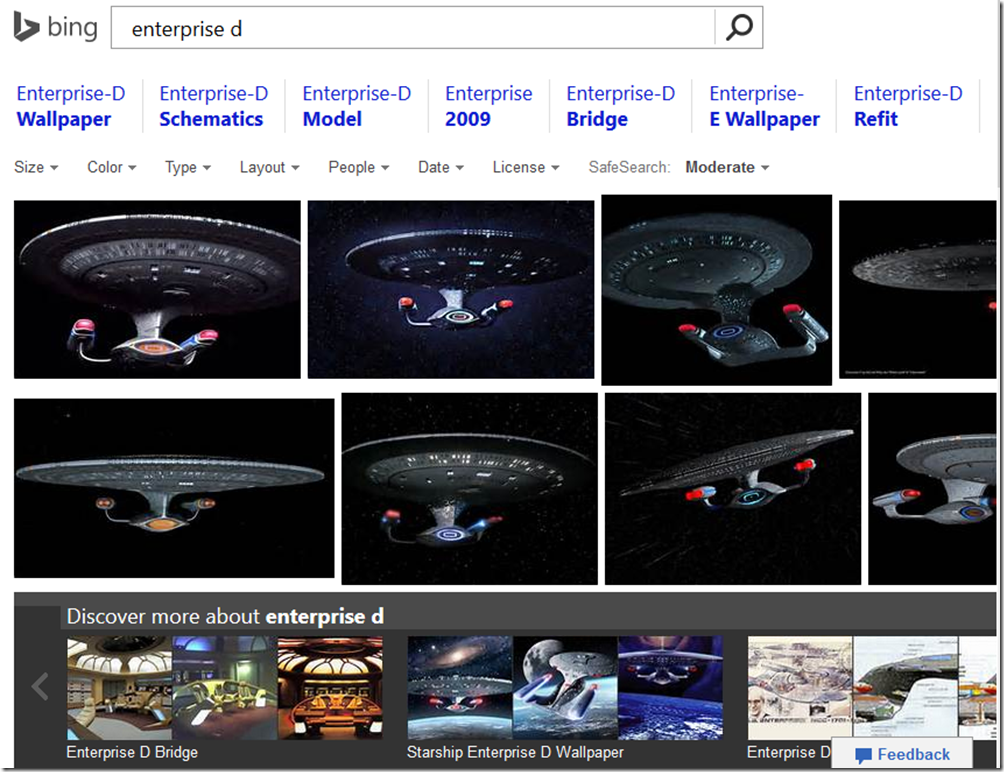
The filtering mechanism even includes niceties like license type:
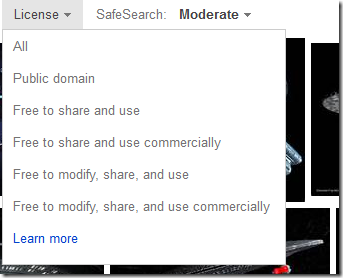
If you’re searching for people, then you can filter by pose as well.
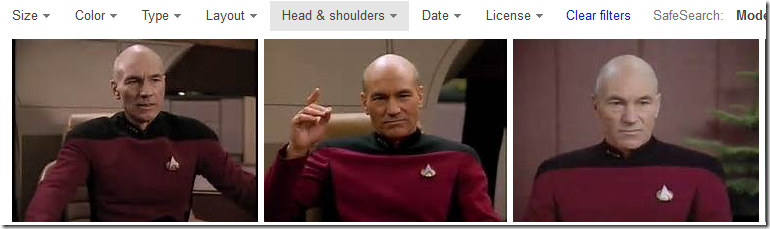
Clicking on an image brings up a pop-up with the larger version of the image. This screen allows you to view the image directly, visit the page it came from, find similar images, Pin it to Pinterest, among other things. Along the bottom is a carousel of the original image search results.
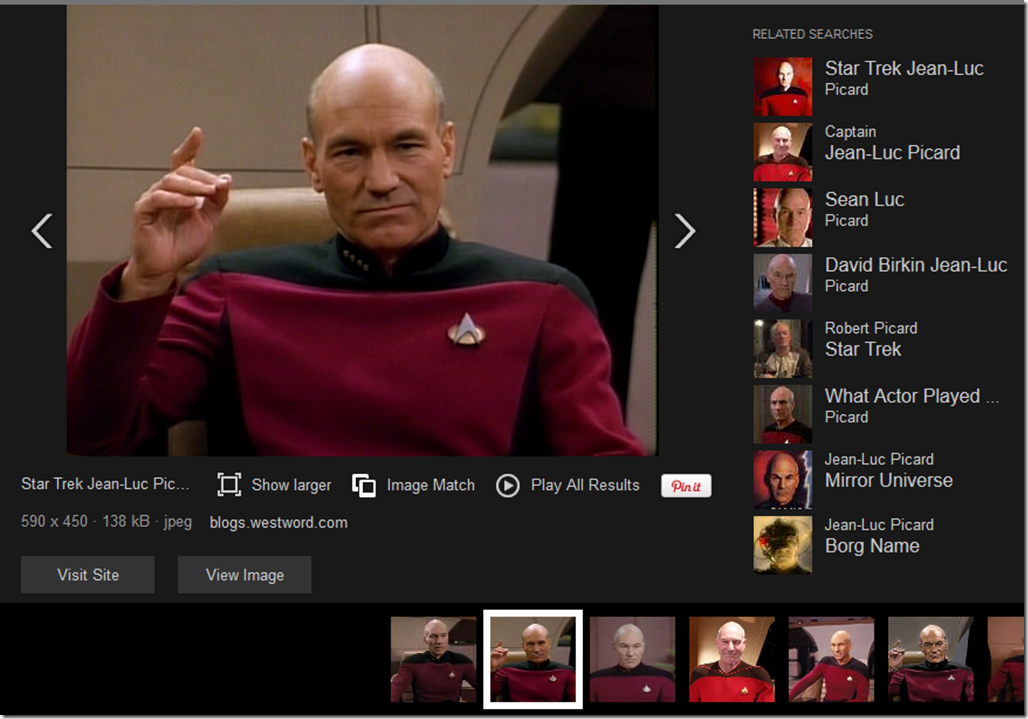
11. Video Search
Like image search, video search is far better than the competition. Holding the mouse over a thumbnail results in a playing preview with sound.
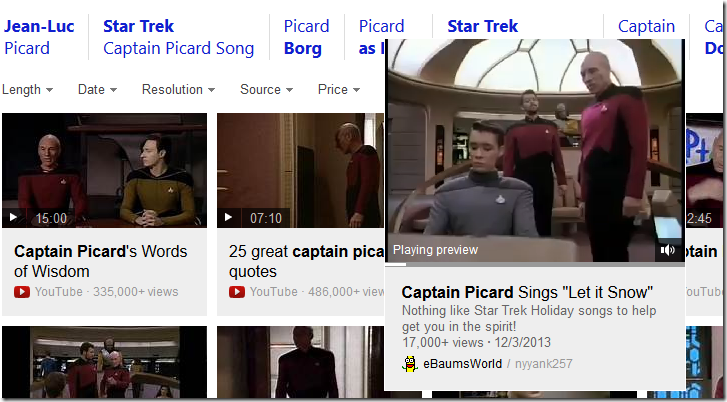
Clicking on a video brings up a larger preview version with a similar layout as the image search.
Like images, the list of videos also has duplicates removed. While YouTube may command the lion’s share of video these days, Bing will show videos from all over the web.
12. It Does Math For You
Yeah, it will do 4+4 for you, and then bring up an interactive calculator:
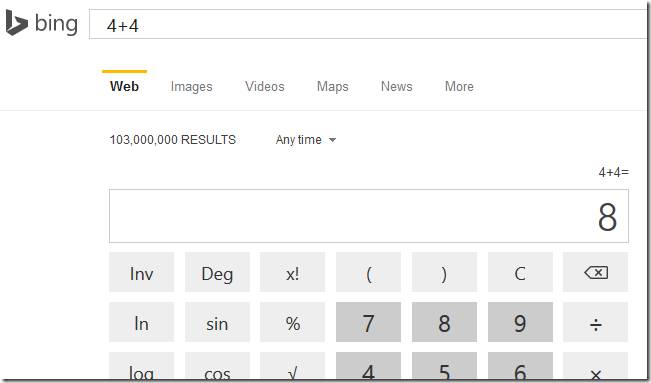
Big. Deal.
However, it can do advanced math too! It can also solve quadratics and other equations:
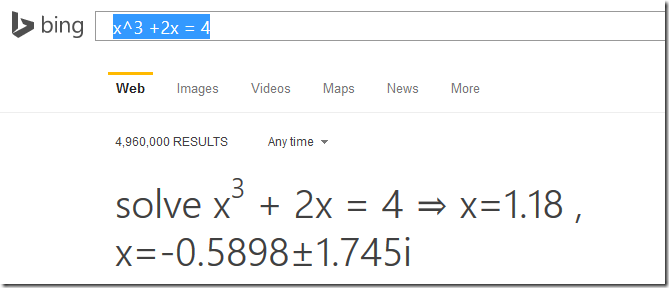
Handles imaginary numbers to boot. Ok, that’s pretty cool. Does the competition do this? No.
13. Unit Conversion
Yes, it will convert all sorts of lengths, areas, volumes, temperatures, and more, even ridiculous ones:
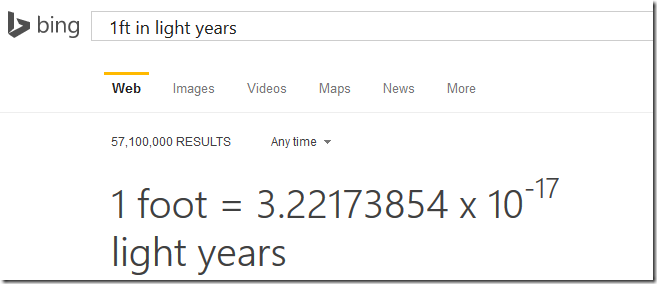
14. Currency Conversion
When I look at my book royalties in Amazon, it displays them in the native currency rather than what I actually care about: good ol’ USD. Bing to the constant rescue:
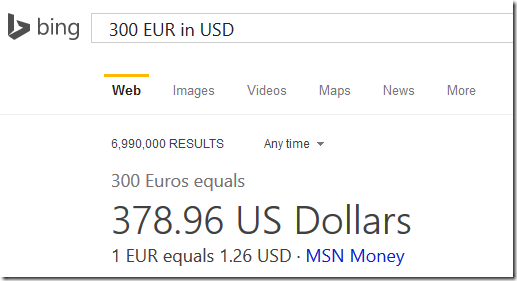
More natural phrasing also works, such as “100 euros in dollars”
15. Finds the Cheapest Flights For You
Searching for: “seattle to los angeles flights” yields this little widget:
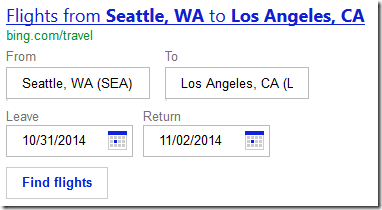
Update your details and click Find flights and you get:
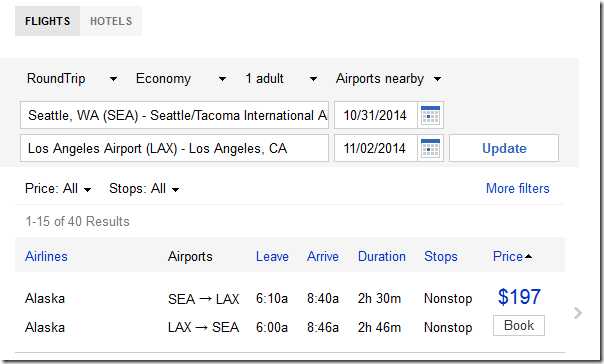
16. Get At-a-Glance University Information
The most useful information about universities is displayed for you right on the results page, including the mailing address, national ranking, enrollment, acceptance rates, tuition, and more, with links to more information.
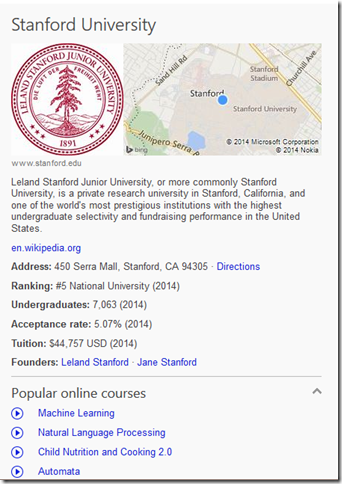
17. Find Online Courses
This is one of the coolest features. Notice the online courses list in the previous image? Those are free, online courses offered by the school. Clicking on them takes you directly the course page where you can sign up.
18. Great Personality and Celebrity Results
You can get a great summary and portal to more information for many, many people. It’s not just limited to the usual actors and recording artists. Atheletes, authors, and more are included too, such as one of my favorite authors, the late, great Robert Jordan (AKA James Oliver Rigney, Jr.):
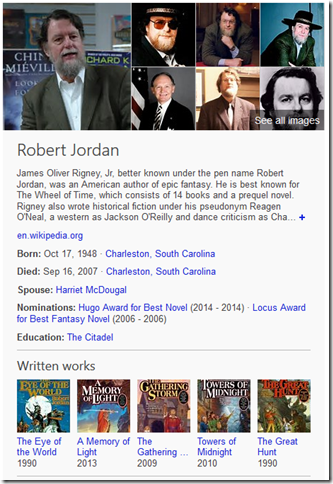
For singers, you can sample some of their tracks right from Bing:
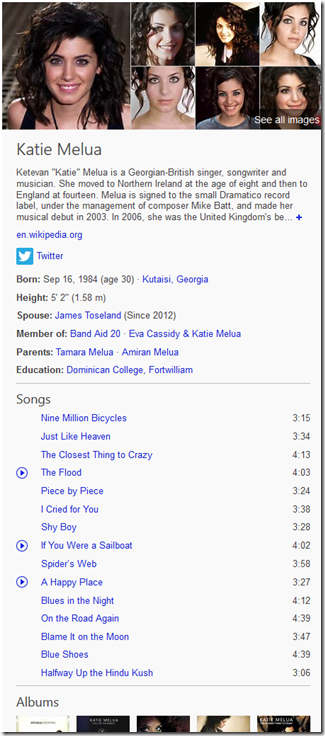
Clicking on a song does another search in Bing which gives more information about the song itself, including lyrics, and links to retailers to purchase the song.
19. Song and Lyric Information
Searching for a song title will give you a sidebar similar to that for artists:
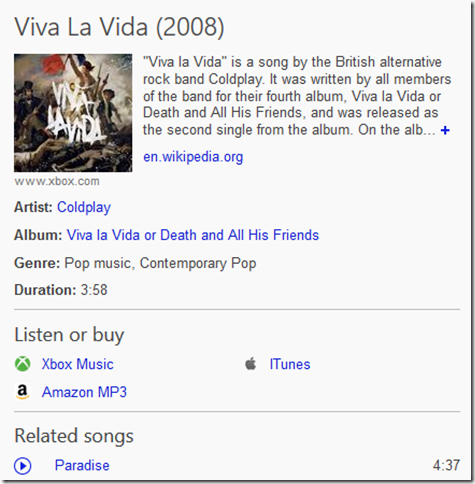
Searching for lyrics specifically will show those as well:
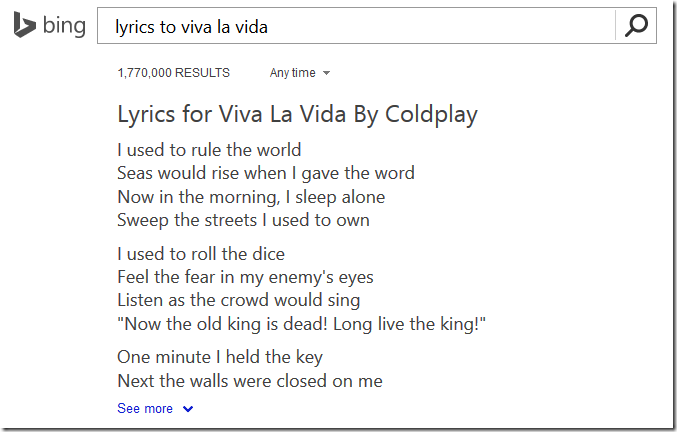
20. Great for Finding “Normals” Too
I don’t have the most popular name (at least in the U.S. – I suspect it’s more common in the U.K.), but if I do a search for it, I get this:
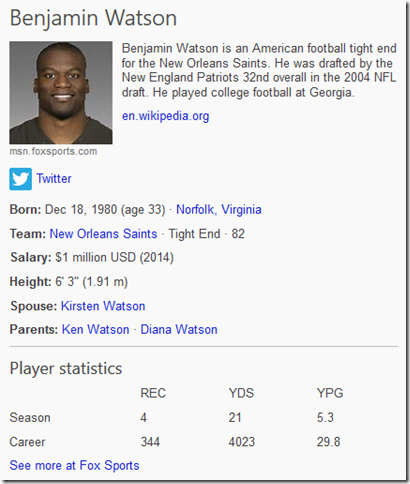
That’s…not me.
But if I qualify my name with my job, “Ben Watson Microsoft Bing”, then I get something about me, admittedly not much (hey, I’m not that famous!):
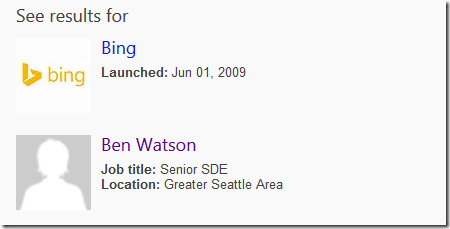
Clicking on my name brings up results including this blog and LinkedIn profile.
21. Find Product Buying Guides
This is probably one of the most popular types of searches. I research things both small and large and while there isn’t always a card for each item, often there is.
Try the search “vacuum cleaner recommendations”:
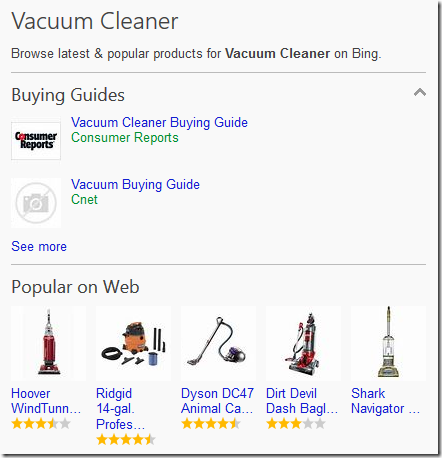
Or better, try “Windows Phone reviews” and you get a similar sidebar:
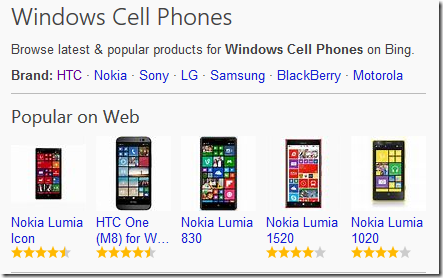
If you click on a manufacturer, it brings up a carousel for phones of that brand:
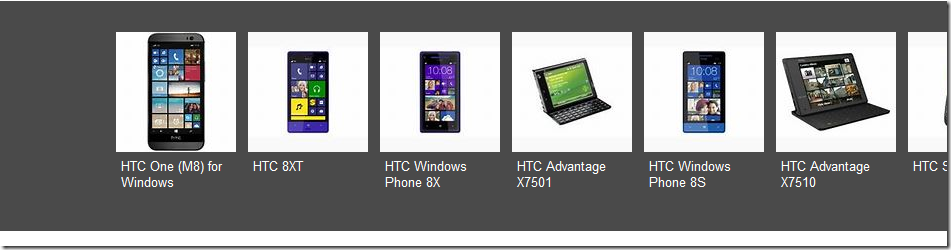
Click on those in turn bring up web search results for each one.
22. The Best Search Engine for Programmers
I have so far abstained from showing many Bing vs. Google head-to-head, but I just have to for this. The query is “GC.WaitForPendingFinalizers”, a .NET Framework method.
Here are the first two results of Bing on the left, compared to Google on the right:
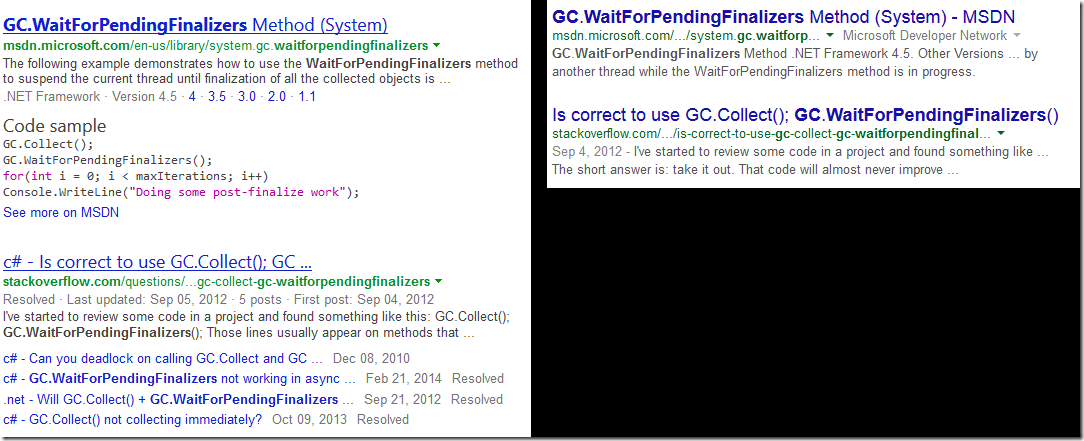
Bing has a much more attractive and useful layout, links to various .NET versions, and a code sample! For the StackOverflow result, it shows related questions grouped under the most relevant question it found.
23. Time Around the World
My family is spread out throughout the world, from all over the US to Europe. I can generally figure out what time it is on the East coast, but what my family in Arizona, where they don’t have Daily Savings Time—are they in the same time zone as me right now or not? What time is it in Sweden?
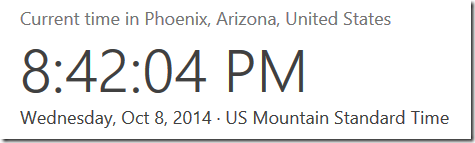
24. Package Tracking
Just copy & paste the tracking number from your product order into Bing, and you’ve got a link directly to the carrier’s tracking page:
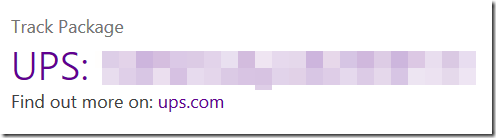
(The tracking number in that screen shot has been censored by me, and there is no link to a live results page.)
25. Search Within the Site, from Bing
Many web sites have search functions built-in to them. You can take advantage of these directly from Bing. For example, search for the popular book social media site Goodreads.com:
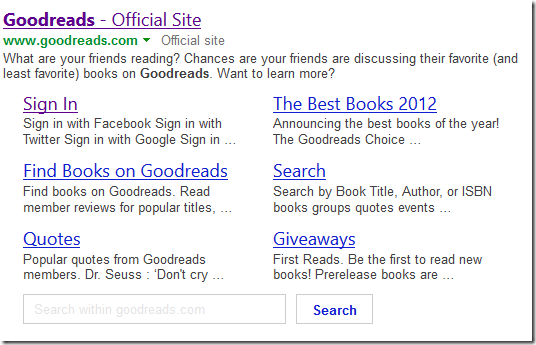
If you then type something into that search back and click “Search” you will be taken to a results page on that web-site directly. Not a huge feature, but it saves you a few clicks.
26. Recipes
You can get top-rated recipes directly in Bing, with enough of a preview to know if you want to read more details.
Here’s a search for “chicken parmesan”:
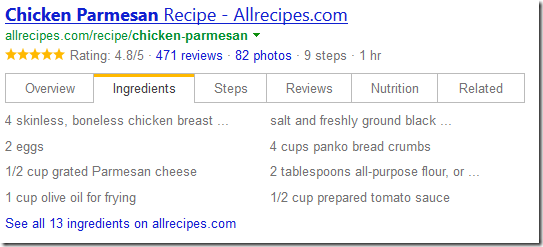
27. Nutritional Information
Highlighted recipes will have nutrition information, but so will plain foods, such as “pork tenderloin”:
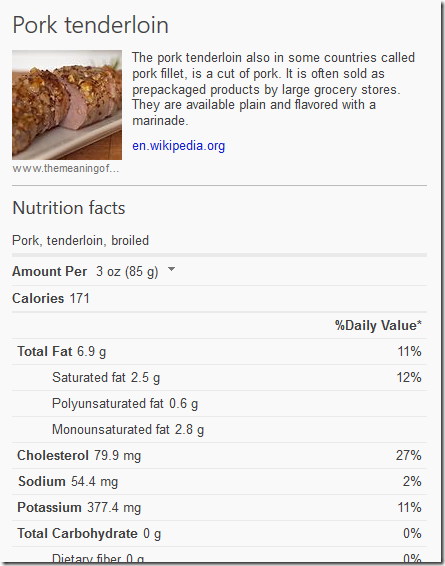
28. Local Searches
The go-to query for this is “pizza”, but around here pho is nearly as important (to me, anyway). If you search for “pho Redmond” you get a carousel showing the top restaurants. This carousel interacts with the map of the local area:
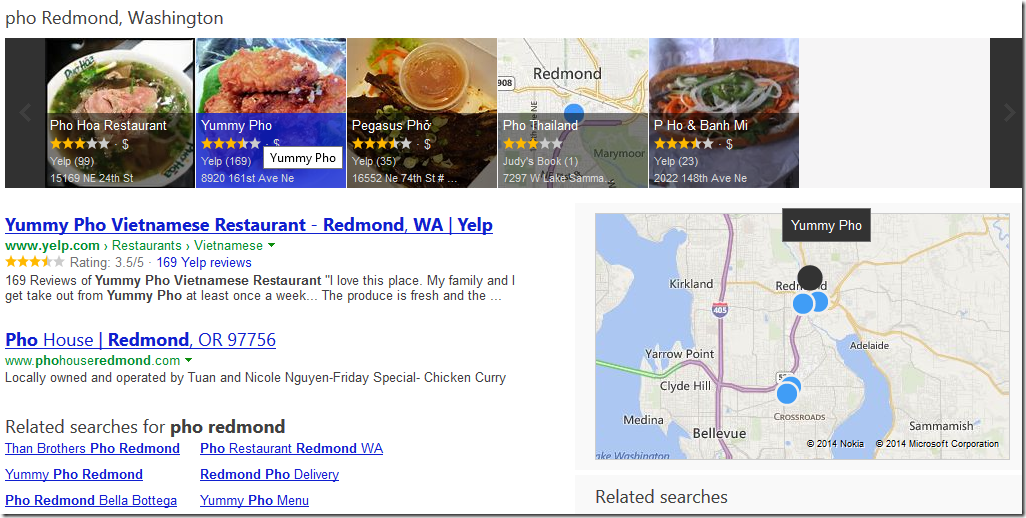
There is an alternate format that shows up for things without pictures, such as piano stores:
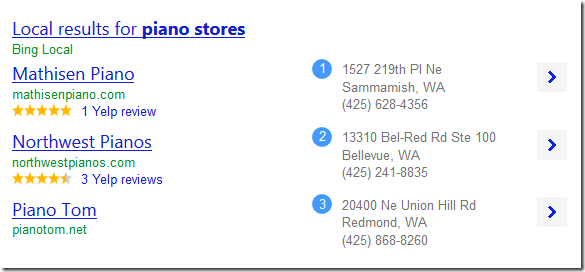
But some topics just have so much about them. Revisiting “pizza”, these results will include the restaurant carousel, nutrition facts, a map, images, and more.
29. City Information
If you do a search for a city, you will get images, some top links for tourist information, and a sidebar containing a map, facts, the current weather, points of interest, and even live webcams!
I lived in Rome for a year, and loved it. There is enough there to fill a month of sight-seeing and still not cover nearly everything. Bing conveys a bit of that:
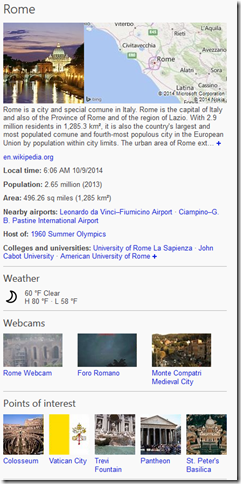
30. Advanced Search Keywords
Sure, Bing tries to guess what you mean just from the words and phrases you type, but there are ambiguous scenarios that require some more finesse. The ones I use more often are “” (quotes), + (must have), and – (must not have). For example:
“Ben Watson” +Bing –football
Indicates that the phrases “Ben Watson” should be included, Bing must be included, and football must NOT be present.
Start with these advanced search options, and then move on to some more advanced keywords that give you even more power, like:
.NET ext:docx
Will find documents ending with the docx extension containing the word .NET.
or site: which restricts results to pages from a specific site, or language: to specify a particular language.
31. Bing Maps and Bird’s Eye View
Bing Maps by itself is great. It provides all the standard features you expect: directions, live traffic, accident notification, satellite imagery, and more.
But the really cool thing is Bird’s Eye View, which offers an up-close, detailed, isomorphic view of an area. Check out this shot:
![image5[1] image5[1]](https://www.philosophicalgeek.com/wp-content/uploads/2014/10/image51.png)
You’ll get that view automatically if you keep zooming in, but you can switch to a standard aerial view as well.
32. Mall Maps
Search for a mall near you and see if it has this information. Here’s one from Tysons Corner Center in Virginia:
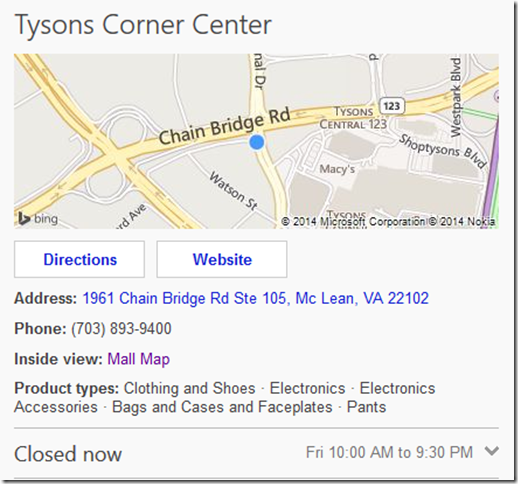
Now click on Mall Map, which will take you to Bing Maps, but show you a map of the inside of the mall!
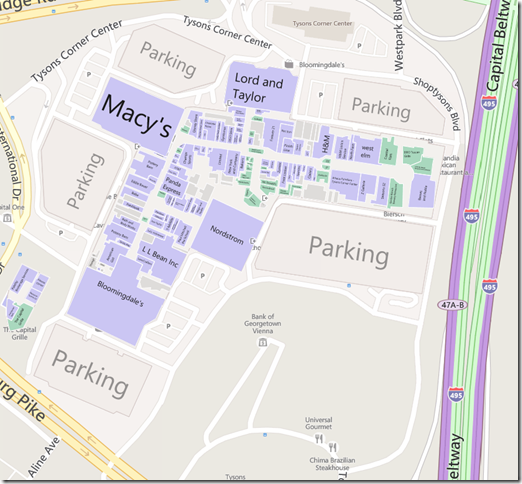
You can even switch levels:
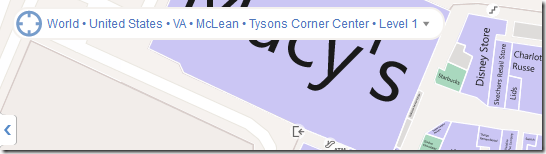
33. Airport Maps
The same inside map feature exists for airports too. Here’s a detail of SeaTac:
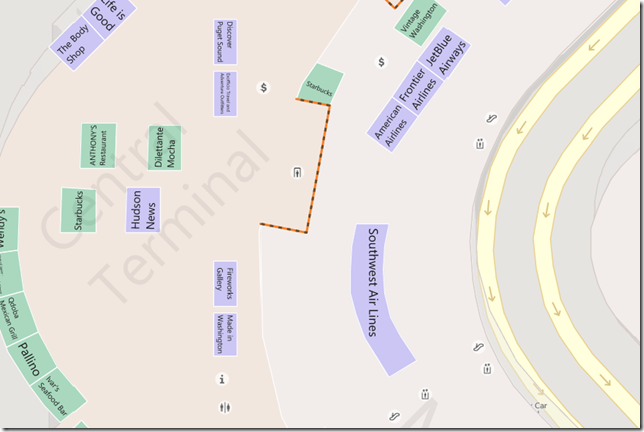
You can see individual carrier counters, escalators, kiosks, stores, and more.
34. Venue Maps
I think you get the idea…
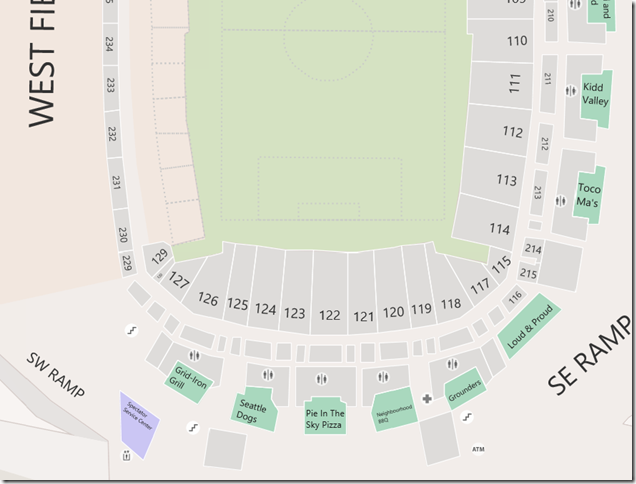
35. Answers Demographic Questions
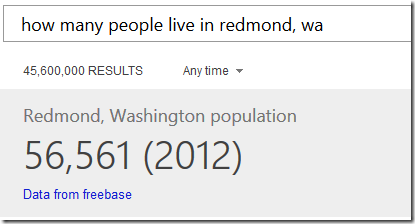
36. Awesome Special Pages
Did you see the Halloween Bing page from 2013? No? I could not find a way to access it now, but someone did put a video of it up:
Bing’s 2013 Halloween home page was interactive
37. Predictions
Bing analyzes historical trends, expert analysis, and popular opinion to predict the outcomes of all sorts of events, including a near-perfect record for the World Cup. Bing can also predict the outcomes of NFL games, Dancing with the Stars, and more.
![image17[2] image17[2]](https://www.philosophicalgeek.com/wp-content/uploads/2014/10/image172.png)
![image22[2] image22[2]](https://www.philosophicalgeek.com/wp-content/uploads/2014/10/image222.png)
To see all of the topics Bing can predict (with more coming soon), head over to http://www.bing.com/explore/predicts.
38. Election Results and Predictions
Bing has had real-time election results for a while, but new this time are predictions. Check it out at http://www.bing.com/elections (or just search for elections). It breaks down results and predictions state-by-state, showing elections for the House, Senate, and Governorship.
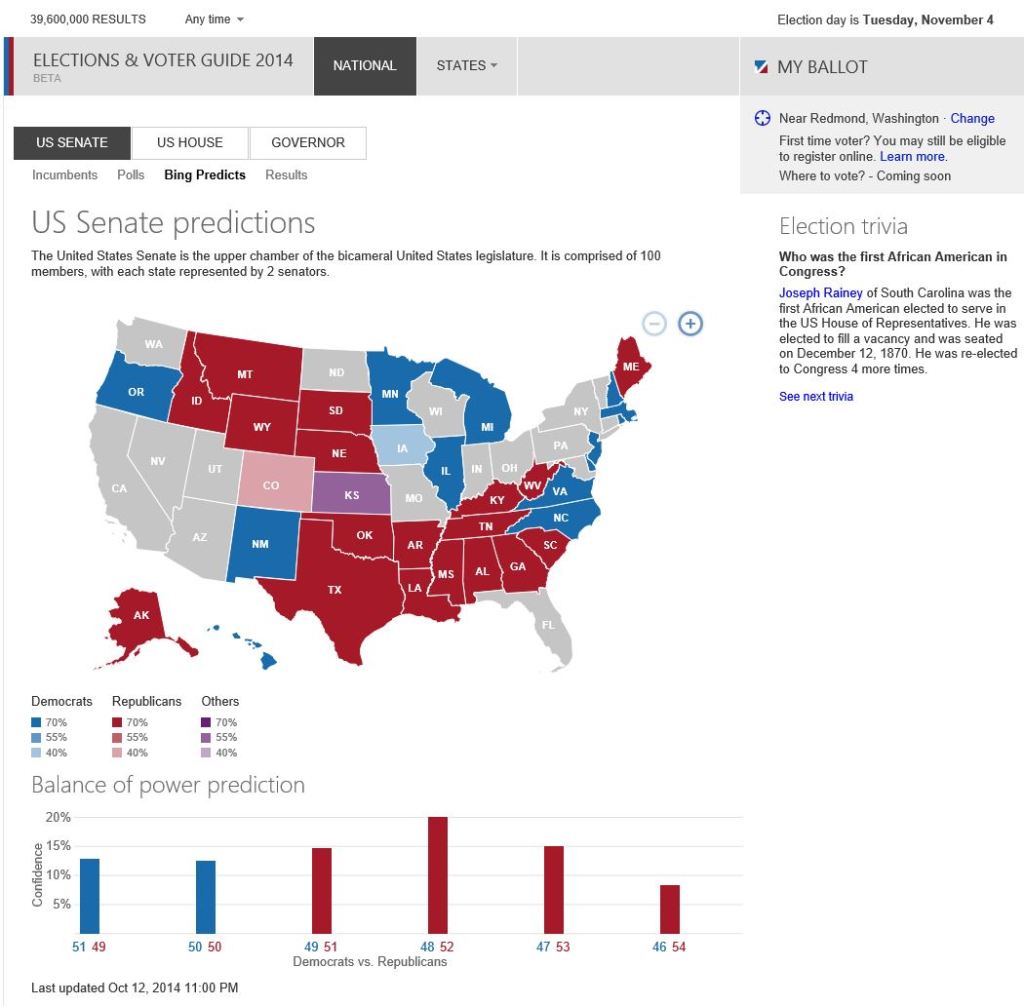
39. Find Seats to Local Events
Go to http://www.bing.com/events to find a list of major events in your area. You can filter by type of event (Music, Sports, etc.), city, date, and distance.
You can even submit your own events!
![image27[1][2] image27[1][2]](https://www.philosophicalgeek.com/wp-content/uploads/2014/10/image2712.png)
40. Language Translation
Bing can translate text for you. For example, I typed the query “translate thank you to italian” and it resulted in:
![image57[1][2] image57[1][2]](https://www.philosophicalgeek.com/wp-content/uploads/2014/10/image5712.png)
You can also go to http://www.bing.com/translator for more control over what you want translated.
Fun fact: Translation on Facebook is done via Bing:
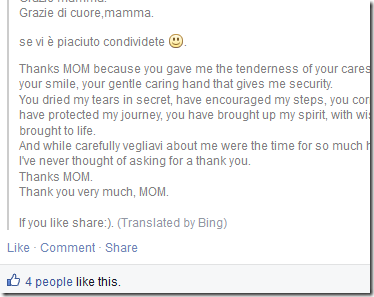
41. Provide feedback on the current query
On the result page, at the bottom, you can provide Feedback about the current query.
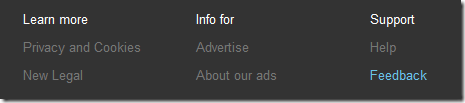
This goes into a database that gets analyzed to suggest improvements for that query, or the system as a whole.
42. Links to Libraries
When you search for a book, you get a great sidebar, similar to that for songs or artists. Besides the usual links to buy the book, you also get a link to your local library to borrow an eBook version.
Other interesting tidbits it gives you are the reading level and other books in the series.
43. Integration Into Windows
Performing a search on your Windows 8 computer shows results from your local computer as well as Bing, all in a seamless, integrated interface. It’s particularly effective on a Surface, with the touch interface.
44. Bing From Xbox One
You can use Bing without a keyboard. Without a mouse. Without a controller. Just your voice! If you have an Xbox One, you can do searches using voice commands, with natural, plain language, e.g., “Show me comedies starring Leslie Nielsen.”
Check out some examples and try them for yourself.
45. Bing is in Office
Bing is integrated into Microsoft Office. You can add Apps into Office that utilize Bing. You do this from the Insert tab on the Ribbon, under My Apps:
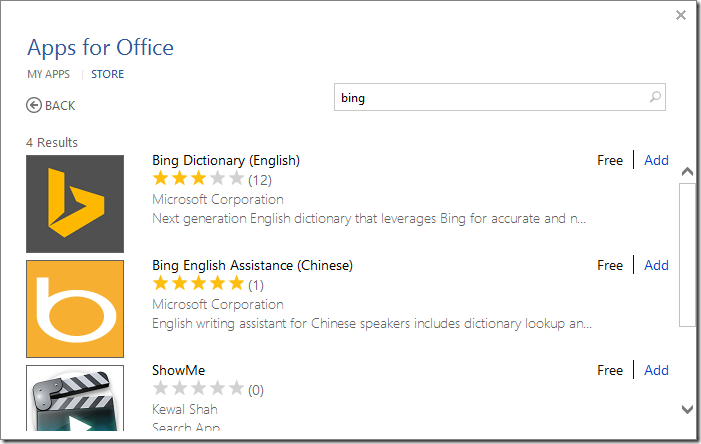
You can also insert images into your document, directly from Bing (via Insert | Online Pictures:
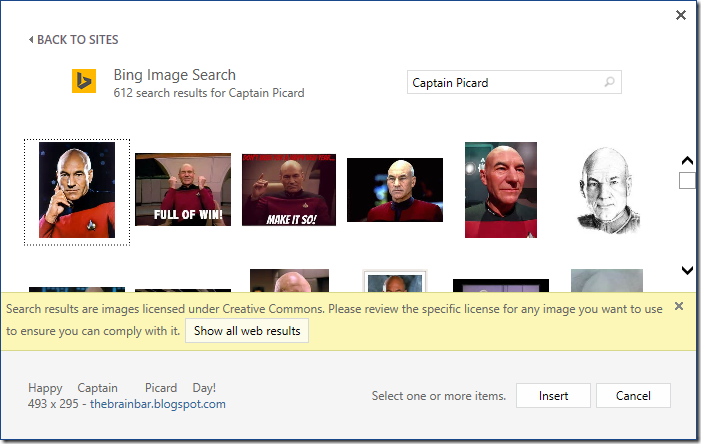
46. Cortana

Cortana is awesome. You can have her record notes for you, remind you to do something at a specific time, search for something, even tell you a corny joke or sing a song.
The best part is that Cortana is powered by Bing and learns from your interests.
If you have Windows Phone, then you should definitely take some time to learn about how you can interact with Cortana.
(Sidebar: Do you wonder who Cortana really is?)
47. Bing Rewards
You can get points for each query you do, for specific tasks, for inviting friends, and more. With the points, you can redeem small prizes. I usually get enough Amazon gift certificates to get something decent every few months.
Go to http://www.bing.com/rewards to sign up, view your status, or redeem the points. Some of the gifts:
- Xbox Live Gold Membership
- Xbox Music Pass
- Amazon gift cards
- Windows Store gift cards
- Skype credits,
- OneDrive storage
- Ad-free Outlook.com
- Flowers
- Restaurants
- Movie tickets
By itself, the program isn’t going to change your life significantly, but it’s a nice little perk.
48. Bing is the Portal for your Microsoft Services
Above the photo, there is a bar with links to the most popular Microsoft destinations, including MSN, Outlook.Com, and Office Online.
![image137[2] image137[2]](https://www.philosophicalgeek.com/wp-content/uploads/2014/10/image1372.png)
49. High-Quality Partners
Microsoft has partnerships with many, many companies to ingest structured data from all over the world in many contexts. Some of the most obvious are Yelp and Trip Advisor. We also have partnerships with Twitter, Apple, Facebook, and many more.
50. Bing is the Portal to Your Day and the World
Yes, Bing is a search engine, and a GREAT one at that, but as demonstrated throughout this entire article, it does a lot more. By organizing and presenting the information in an attractive format, it aspires to be a lot more than just 10 blue links. You can learn and grow, find your information faster, explore related topics, answer your questions, and solve more problems. Bing is for people who want to experience the world.
It looks better, it performs better, it is awesome.